Learn & Record
Android (갤러리, 스피너, 구글 지도, 구글 클라우드, API 연동, 아이콘 생성 ) 본문
1. 갤러리
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<Gallery
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/gallery"
android:spacing="5dp"/>
<ImageView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/imageViewPoster"
android:padding="20dp"/>
</LinearLayout>
package kr.jeongmo.a0513_project
import android.content.Context
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.view.View
import android.view.ViewGroup
import android.widget.BaseAdapter
import android.widget.Gallery
import android.widget.ImageView
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
title = "갤러리 영화 포스터"
val gallery = findViewById<Gallery>(R.id.gallery)
val myGalleryAdapter = MyGalleryAdapter(this)
gallery.adapter = myGalleryAdapter
}
inner class MyGalleryAdapter(val context: Context): BaseAdapter() {
val posterId = arrayOf(
R.drawable.mov01,R.drawable.mov02,R.drawable.mov03,
R.drawable.mov04,R.drawable.mov05,R.drawable.mov06,
R.drawable.mov07,R.drawable.mov08,R.drawable.mov09,
R.drawable.mov10,R.drawable.mov11,R.drawable.mov12,
)
override fun getCount(): Int {
return posterId.size
}
override fun getItem(p0: Int): Any {
return 0
}
override fun getItemId(p0: Int): Long {
return 0
}
override fun getView(p0: Int, p1: View?, p2: ViewGroup?): View {
val imageView = ImageView(context)
imageView.layoutParams = Gallery.LayoutParams(200,300)
imageView.scaleType = ImageView.ScaleType.FIT_CENTER
imageView.setPadding(5,5,5,5)
imageView.setImageResource(posterId[p0])
return imageView
}
}
}
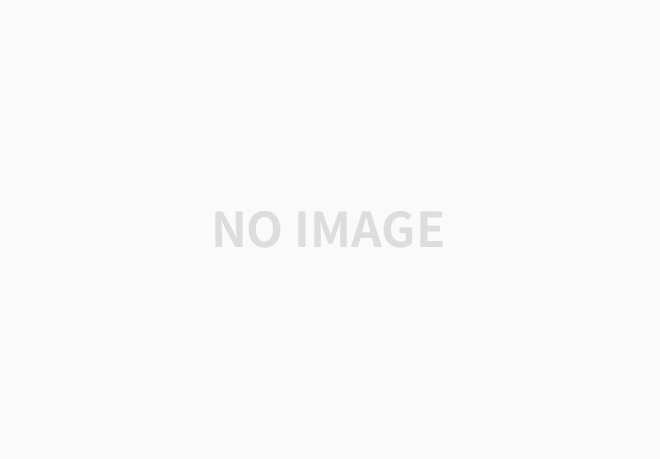
override fun getView(p0: Int, p1: View?, p2: ViewGroup?): View {
val imageView = ImageView(context)
imageView.layoutParams = Gallery.LayoutParams(200,300)
imageView.scaleType = ImageView.ScaleType.FIT_CENTER
imageView.setPadding(5,5,5,5)
imageView.setImageResource(posterId[p0])
imageView.setOnTouchListener { v, event ->
val imageViewPoster = findViewById<ImageView>(R.id.imageViewPoster)
imageViewPoster.scaleType = ImageView.ScaleType.FIT_CENTER
imageViewPoster.setImageResource(posterId[p0])
false
}
return imageView
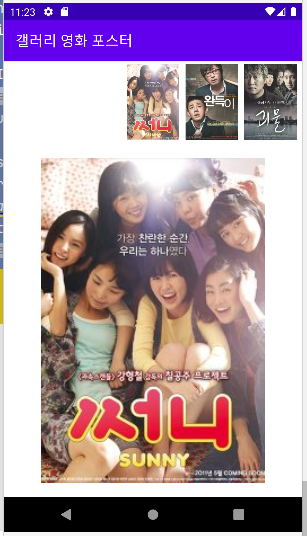
2. 스피너
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Spinner
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/spinner"/>
</LinearLayout>
package kr.jeongmo.a0513_project_spinner
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.ArrayAdapter
import android.widget.Spinner
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
title = "스피너 테스트"
val movie = arrayOf("써니", "완득이", "괴물", "라디오 스타", "비열한 거리",
"왕의 남자", "아일랜드", "웰컴 투 동막골", "헬보이", "백 투더 퓨처")
val spinner = findViewById<Spinner>(R.id.spinner)
val adapter: ArrayAdapter<String> =
ArrayAdapter(this, android.R.layout.simple_spinner_item,movie)
spinner.adapter = adapter
}
}
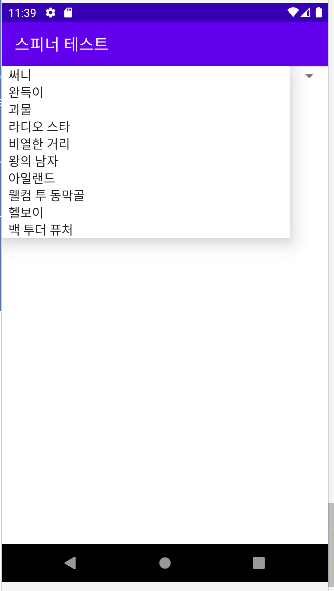
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<Spinner
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/spinner"/>
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="20dp"/>
</LinearLayout>
package kr.jeongmo.a0513_project_spinner
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.view.View
import android.widget.AdapterView
import android.widget.ArrayAdapter
import android.widget.ImageView
import android.widget.Spinner
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
title = "스피너 테스트"
val movie = arrayOf("써니", "완득이", "괴물", "라디오 스타", "비열한 거리",
"왕의 남자", "아일랜드", "웰컴 투 동막골", "헬보이", "백 투더 퓨처")
val spinner = findViewById<Spinner>(R.id.spinner)
val adapter: ArrayAdapter<String> =
ArrayAdapter(this, android.R.layout.simple_spinner_item,movie)
spinner.adapter = adapter
val posterID = arrayOf(
R.drawable.mov01,R.drawable.mov02,R.drawable.mov03,R.drawable.mov04,
R.drawable.mov05,R.drawable.mov06,R.drawable.mov07,R.drawable.mov08,
R.drawable.mov09,R.drawable.mov10
)
spinner.onItemSelectedListener = object: AdapterView.OnItemSelectedListener {
override fun onItemSelected(p0: AdapterView<*>?, p1: View?, p2: Int, p3: Long) {
val imageView = findViewById<ImageView>(R.id.imageView)
imageView.scaleType = ImageView.ScaleType.FIT_CENTER
imageView.setPadding(5,5,5,5)
imageView.setImageResource(posterID[p2])
}
override fun onNothingSelected(p0: AdapterView<*>?) {
}
}
}
}
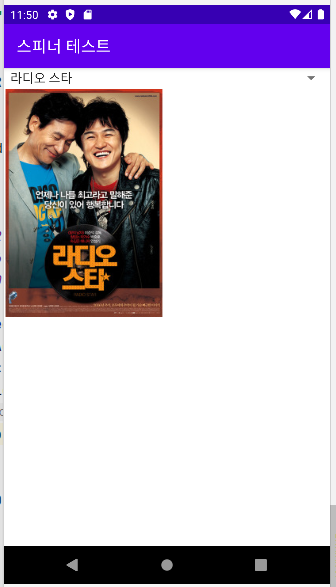
3. 구글 지도
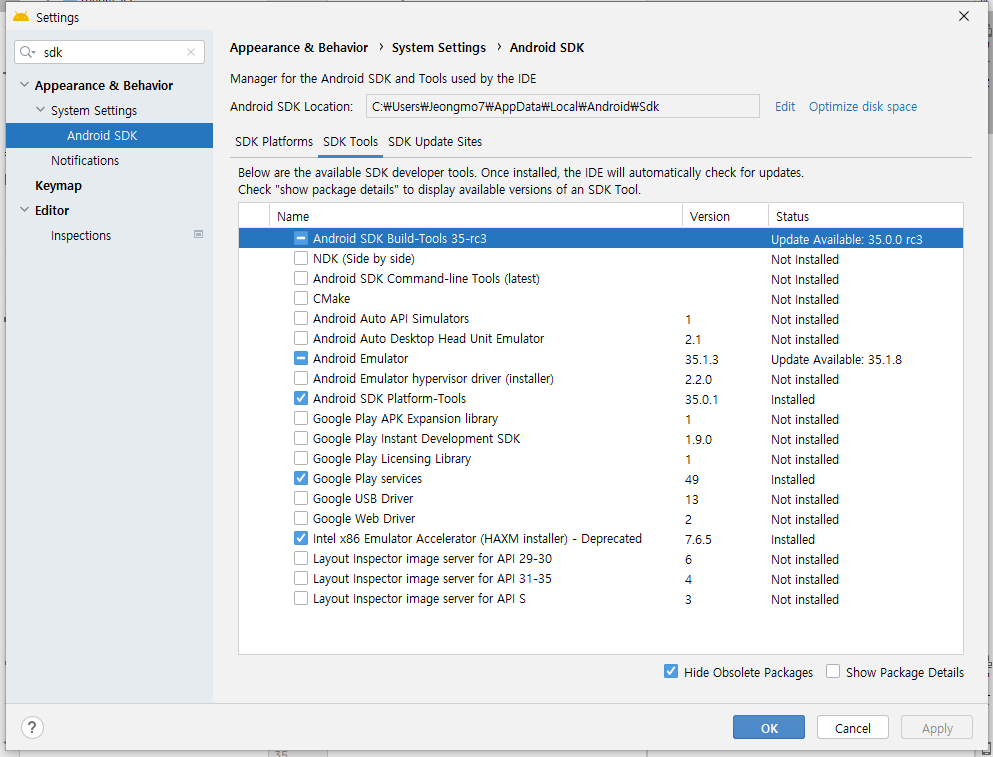
- Google Play services 체크 확인
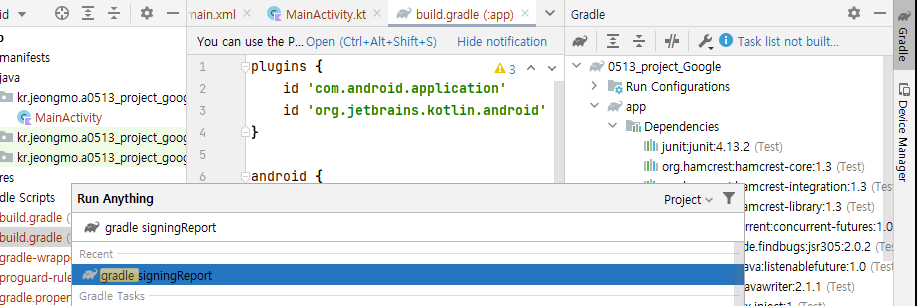
- 오른쪽 탭 Gradle > 코끼리 아이콘 > gradle signingReport 작성 후 실행
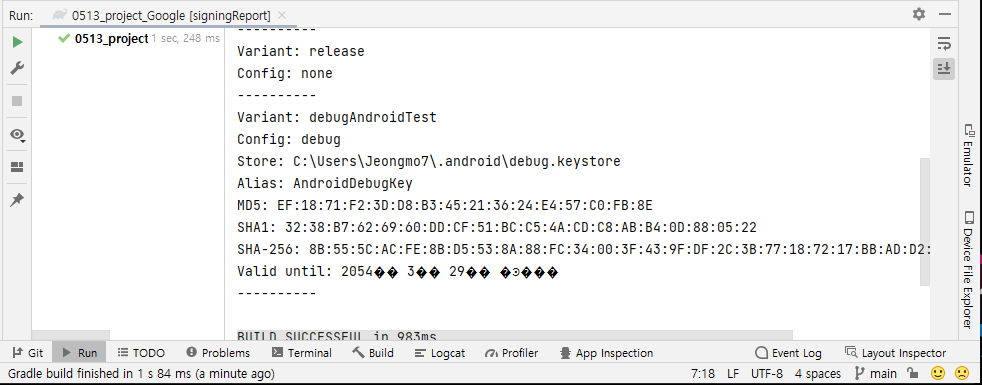
- 확인

- 패키지명 확인
클라우드 컴퓨팅 서비스 | Google Cloud
데이터 관리, 하이브리드 및 멀티 클라우드, AI와 머신러닝 등 Google의 클라우드 컴퓨팅 서비스로 비즈니스 당면 과제를 해결하세요.
cloud.google.com
- 이동 > 계정 생성
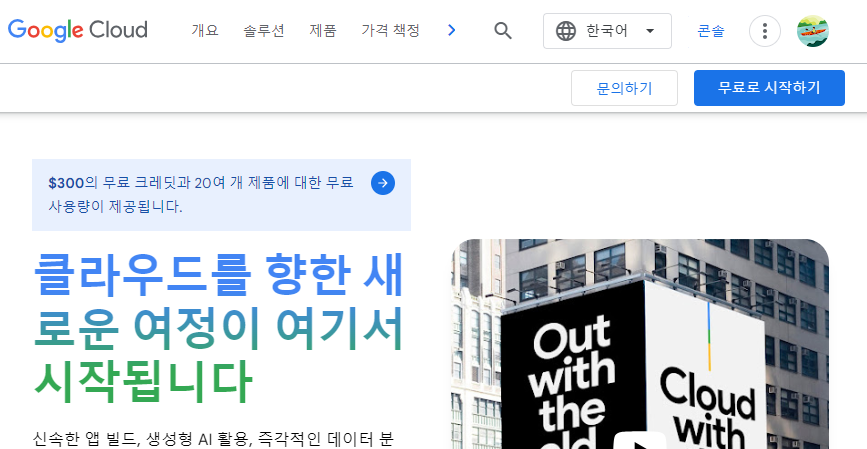
- 접속 후 [콘솔] 클릭
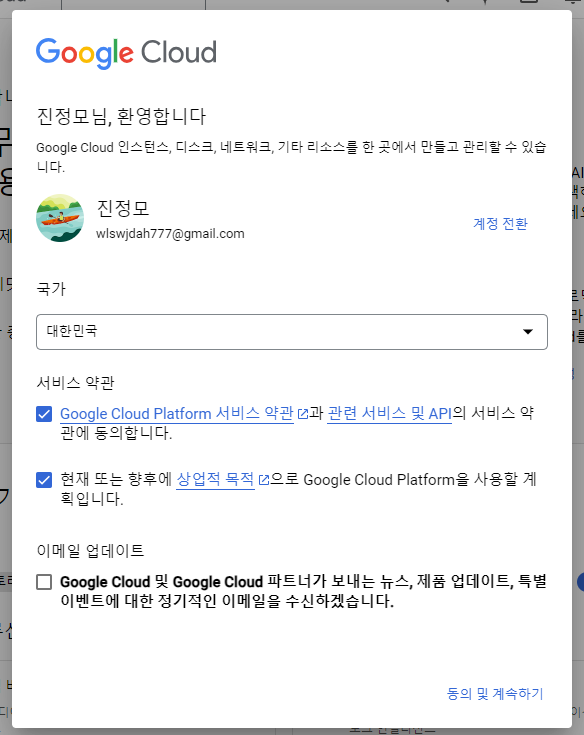
- 약관 동의
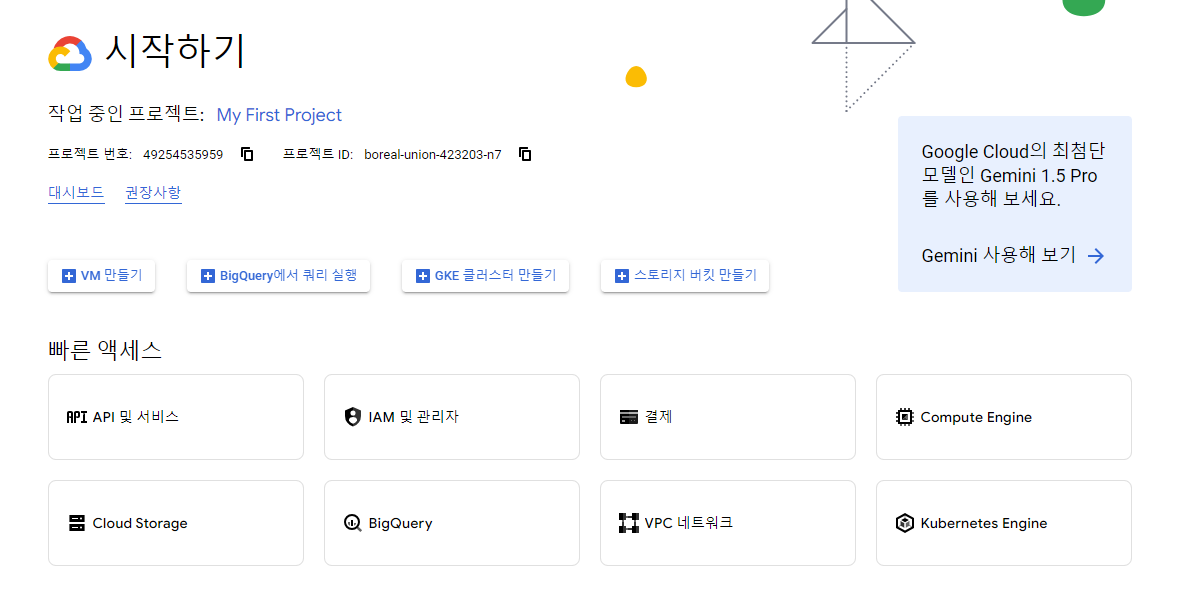
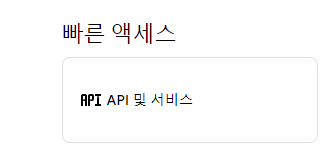
- 콘솔에서 API 및 서비스 클릭
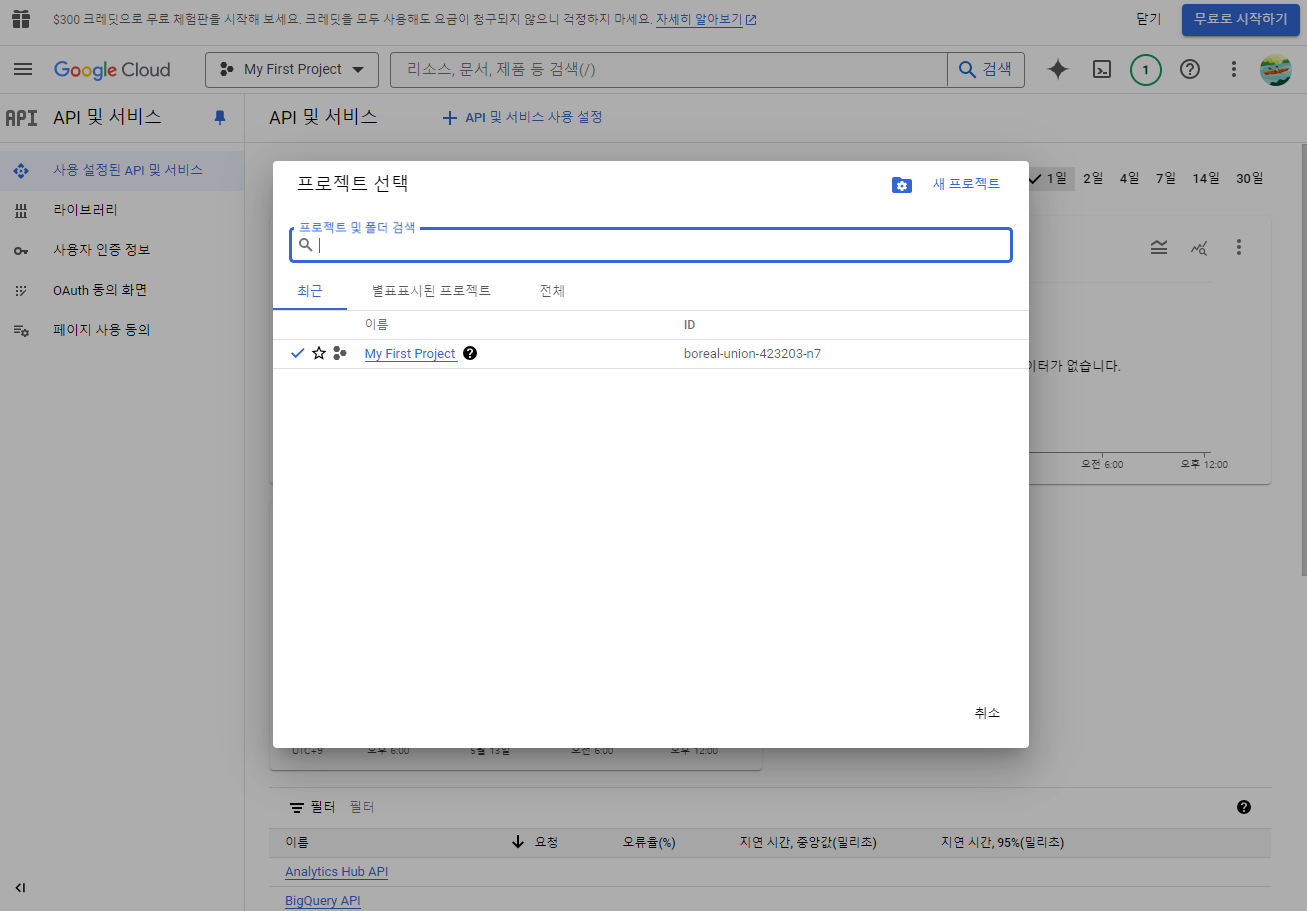
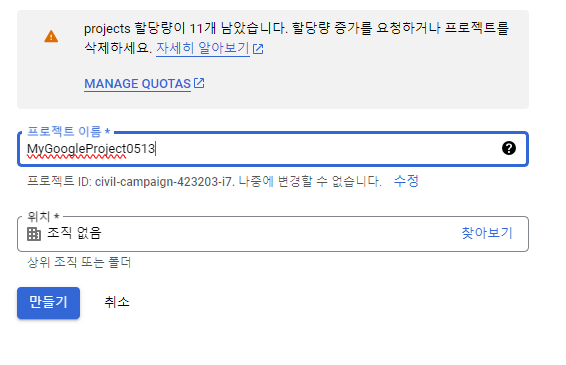
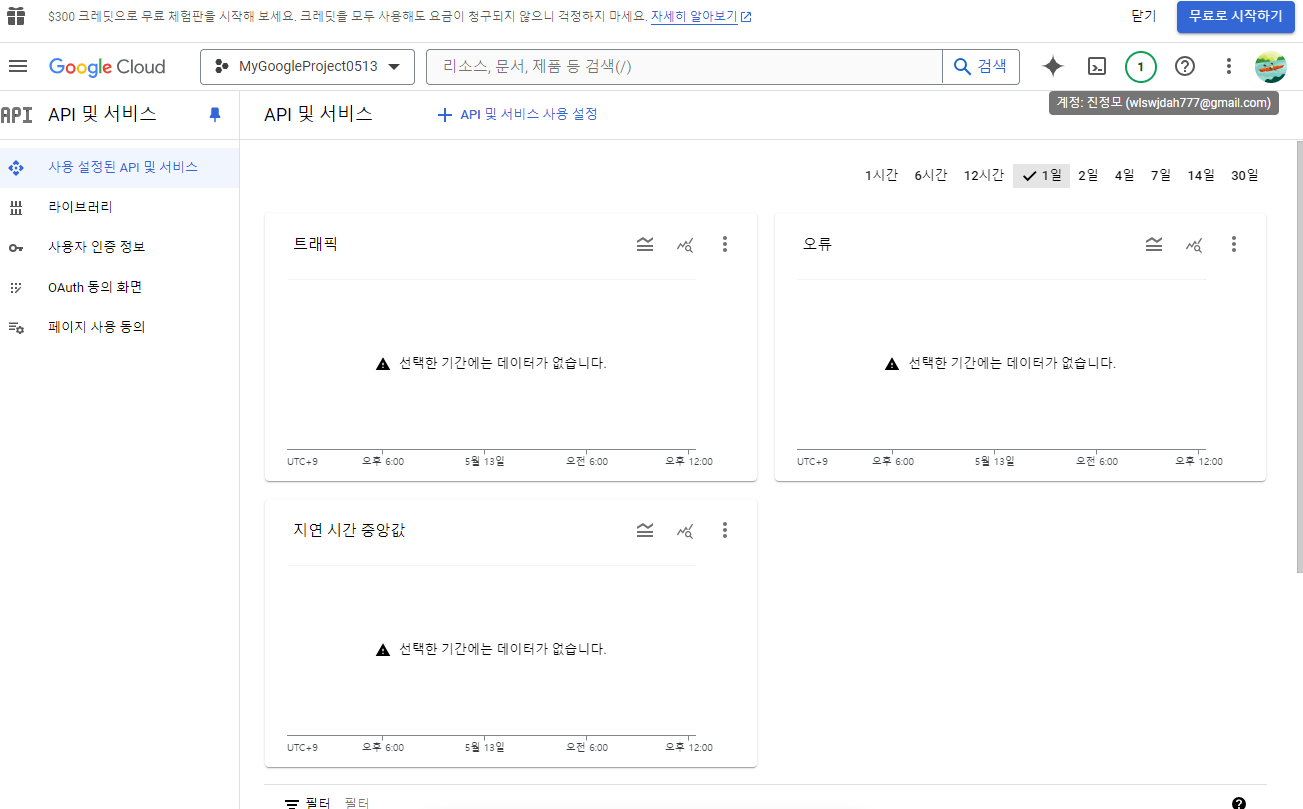
- 알아보기 쉬운 프로젝트명으로 하고 프로젝트 생성

- 버튼 클릭
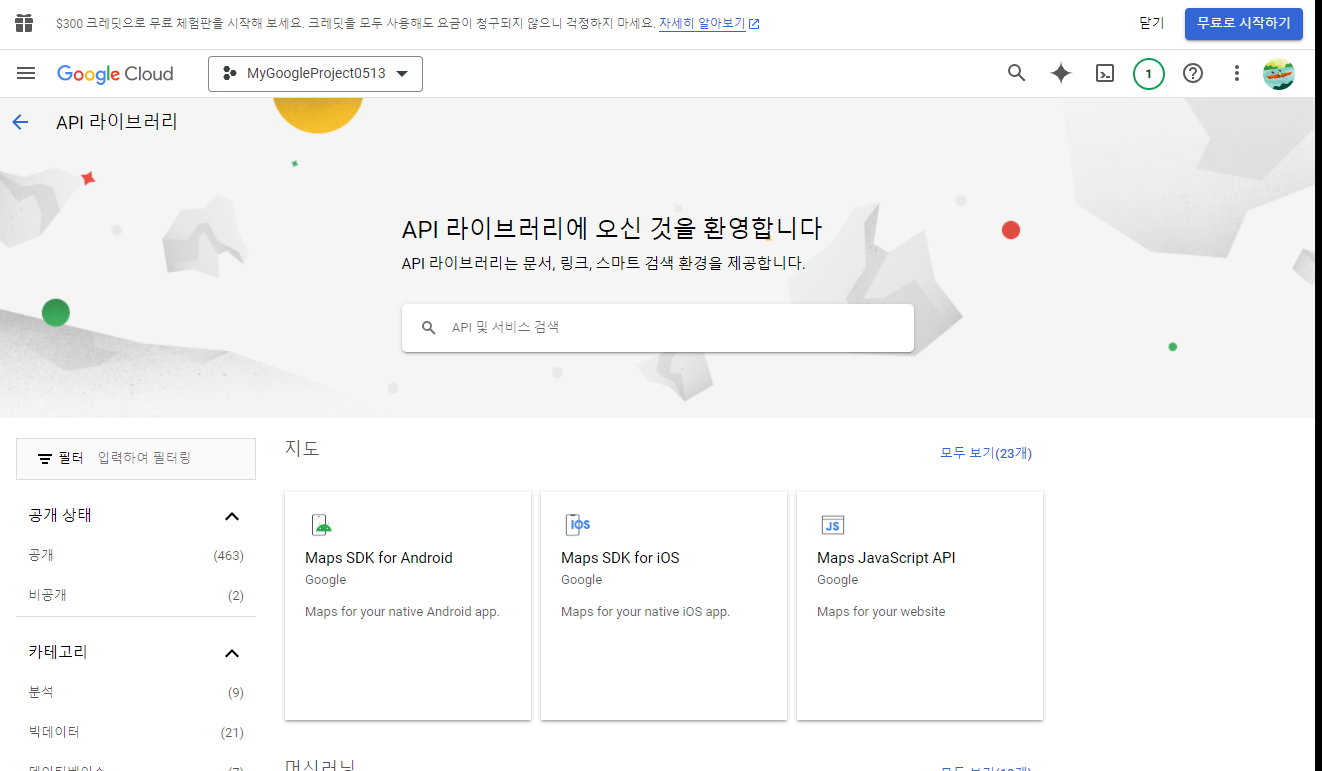
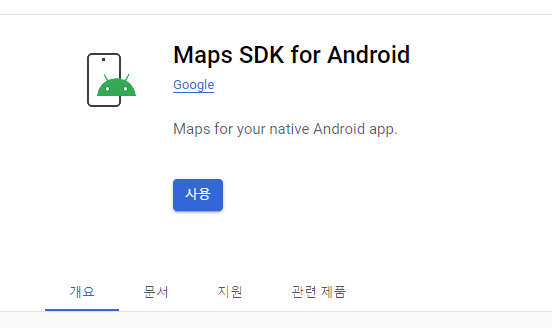
- 아이콘 누른 뒤 > 사용 클릭
- 결제 수단 입력 후 > 무료로 시작하기 클릭
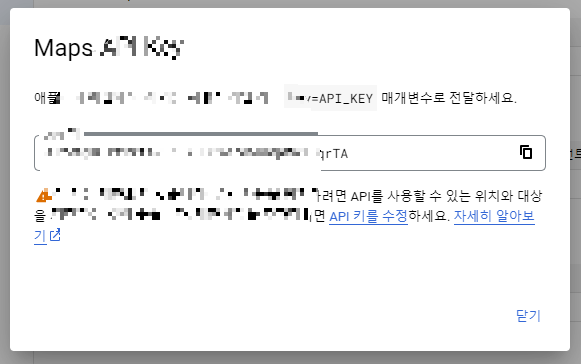
- API 키 복사 해둘 것
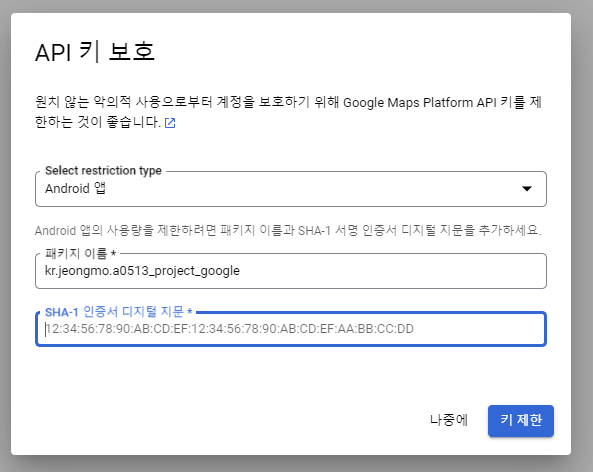
- 키보호 하기
- SHA-1 인증서 디지털 지문 : Gradle signingReport 실행하면 확인 가능
// Google Map
implementation('com.google.android.gms:play-services-maps:17.0.0')
implementation('com.google.android.gms:play-services-location:17.0.0')
- build.gradle에 위 코드 2줄 추가
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="kr.jeongmo.a0513_project_google">
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION"/>
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.0513_project_Google">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<!-- 구글 플레이 서비스 버전을 적은 것으로 그대로 타이핑 -->
<meta-data android:name="com.google.android.gms.version" android:value="@integer/google_play_services_version" />
<!-- 안드로이드 파이 버전부터 필요 -->
<uses-library android:name="org.apache.http.legacy" android:required="false"/>
<!-- 구글 클라우드 플랫폼에서 발급받은 API 키 -->
<meta-data android:name="com.google.android.maps.v2.API_KEY" android:value="구글클라우드 API키"/>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<fragment xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:map="http://schemas.android.com/apk/res-auto"
android:id="@+id/map"
android:name="com.google.android.gms.maps.MapFragment"
android:layout_width="match_parent"
android:layout_height="match_parent"
map:cameraTargetLat="35.86952722"
map:cameraTargetLng="128.6061745"
map:cameraZoom="15"/>
- activity_main.xml에 위 코드 작성
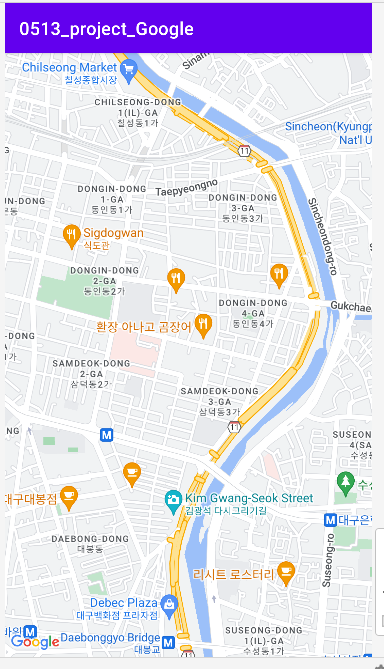
package kr.jeongmo.a0513_project_google
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.view.Menu
import android.view.MenuItem
import com.google.android.gms.maps.*
import com.google.android.gms.maps.model.LatLng
class MainActivity : AppCompatActivity(), OnMapReadyCallback {
lateinit var googleMap: GoogleMap
lateinit var mapFragment: MapFragment
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
title = "Google 지도 활용"
mapFragment = fragmentManager.findFragmentById(R.id.map) as MapFragment
mapFragment.getMapAsync(this)
}
override fun onMapReady(p0: GoogleMap?) {
googleMap = p0!!
// googleMap.mapType = GoogleMap.MAP_TYPE_SATELLITE
// googleMap.moveCamera(CameraUpdateFactory
// .newLatLngZoom(LatLng(35.86952722, 128.6061745), 15f))
}
override fun onCreateOptionsMenu(menu: Menu): Boolean {
super.onCreateOptionsMenu(menu)
menu.add(0, 1, 0, "위성 지도")
menu.add(0, 2, 0, "일반 지도")
menu.add(0, 3, 0, "월드컵경기장 바로가기")
return true
}
override fun onOptionsItemSelected(item: MenuItem): Boolean {
when (item.itemId) {
1 -> {
googleMap.mapType = GoogleMap.MAP_TYPE_HYBRID
return true
}
2 -> {
googleMap.mapType = GoogleMap.MAP_TYPE_NORMAL
return true
}
3 -> {
googleMap.moveCamera(
CameraUpdateFactory
.newLatLngZoom(LatLng(35.86952722, 128.6061745), 15f)
)
return true
}
}
return true
}
}
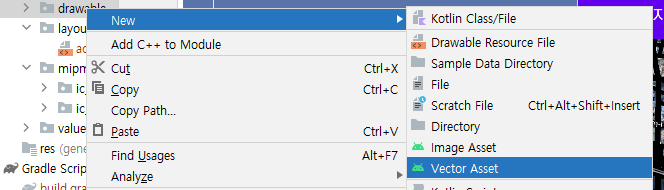
- drawable > New > Vector Asset 클릭
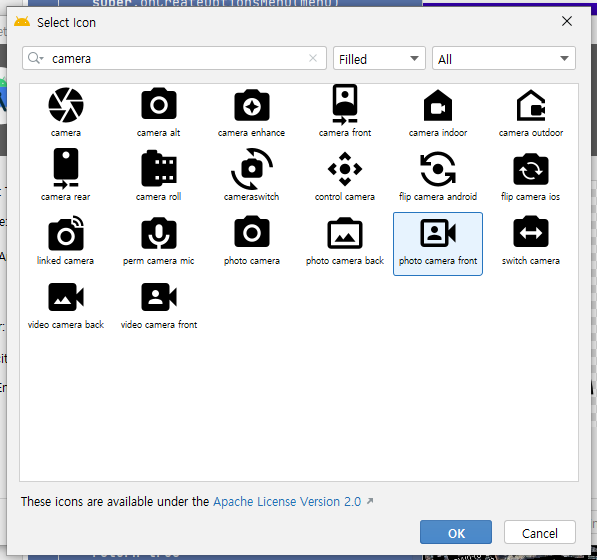
- camera 검색
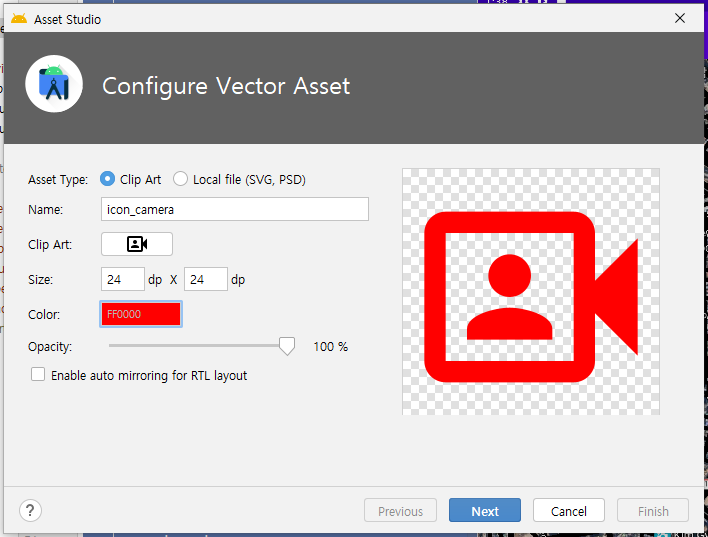
- 이름 변경, 색상 변경 > next > finish
공부 과정을 정리한 것이라 내용이 부족할 수 있습니다.
부족한 내용은 추가 자료들로 보충해주시면 좋을 것 같습니다.
읽어주셔서 감사합니다 :)
'Dev > Android' 카테고리의 다른 글
[Android 오류_해결 5가지] the emulator process for avd has terminated (2) | 2024.05.22 |
---|---|
Android (어댑터뷰, 리스트뷰, 동적으로 항목 추가, 그리드뷰) (0) | 2024.05.10 |
Android (액티비티 생명주기, 로그캣, 액티비티 테스트, 안드로이드 4대 구성요소, 디자인탭 활용, 액티비티와 프래그먼트) (0) | 2024.05.09 |
Android (양방향 액티비티, 암시적 인텐트, 액티비티와 인텐트) (0) | 2024.05.08 |
Android (명화 선호도 투표 프로젝트 기능 추가 ) (0) | 2024.05.07 |