Learn & Record
JavaScript (인천국제공항_버스정보_ 공공데이터 활용, 여객편 주간 운항 공공데이터) 본문
1. 인천국제공항_버스정보_ 공공데이터 활용
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
table {
border: 2px solid black;
empty-cells: hide;
width: 1000px;
table-layout: fixed;
word-break: break-all;
}
td {
width: 200px;
height: 40px; /* 셀의 높이 */
padding: 15px;
border: 1px solid black;
text-align: center;
}
div {
margin: 0 auto;
padding: 20px;
text-align: center;
width: 1000px;
}
div input {
width: 70px;
height: 40px;
background-color: #3a4bb9;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
padding: 5px 10px;
}
div input:hover {
background-color: white;
color: #3a4bb9;
}
</style>
<script>
// 이벤트 등록
document.addEventListener('DOMContentLoaded', function (){
const btns = document.querySelectorAll('input[type=button]')
btns.forEach((item) => {
item.addEventListener('click', function () {
console.log(item.value)
console.log(getUrl())
printBusTable(getUrl(), item.value)
})
})
});
const sortStr = function (string1, string2) {
// 기본 데이터는 문자열. 2개의 문자열을 결합하고, ',' 기준으로 배열로 변환
let tempList = (string1+', '+string2).split(",")
tempList = [...new Set(tempList)] //중복 제거
temlList = tempList.map((item) => item.trim()) // 공백 제거
tempList.sort // 정렬
// 현재 시간 구해서 이전 시간이면 연하게 출력.
const today = new Date();
const todayTime = `${today.getHours()}${today.getMinutes()}`;
// console.log(todayTime)
tempList = tempList.map((item) => {
console.log(Number(item))
return Number(item) < Number(todayTime) ? `<span style="color: #cccccc">${item}</span>` : item;
});
return tempList.join(", ") ; // 배열을 문자열로 변환
}
const getUrl = function () {
const serviceKey = ''
const url = 'http://apis.data.go.kr/B551177/BusInformation/getBusInfo';
return `${url}?serviceKey=${serviceKey}&area=6&numOfRows=50&pageNo=1&type=json`
}
const printBusTable = function (url, name) {
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.onreadystatechange = () => {
if (xhr.readyState === XMLHttpRequest.DONE) return;
if (xhr.status === 200) {
const jsonObject = JSON.parse(xhr.response)
const items = jsonObject.response.body.items;
const tableBody = document.querySelector('tbody');
while (tableBody.firstChild) {
tableBody.removeChild(tableBody.firstChild);
}
for (item of items) {
// console.log(item)
if (item['routeinfo'].indexOf(name) >= 0) {
const trTag = document.createElement('tr')
trTag.innerHTML = `
<td>${item['busnumber']}</td>
<td>${item['busclass']}</td>
<td>${item['adultfare']}</td>
<td>${sortStr(item['t1wdayt'],item['t2wdayt'])}</td>
<td>${sortStr(item['t1wt'],item['t2wt'])}</td>
`;
tableBody.append(trTag)
}
}
} else {
console.error('Error', xhr.status, xhr.statusText)
}
}
xhr.send();
}
</script>
</head>
<body>
<table>
<thead>
<tr>
<th>버스번호</th>
<th>버스등급</th>
<th>성인요금</th>
<th>평일시간표</th>
<th>주말시간표</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
<div>
<input type="button" value="대구">
<input type="button" value="구미">
<input type="button" value="경산">
<input type="button" value="포항">
</div>
</body>
</html>
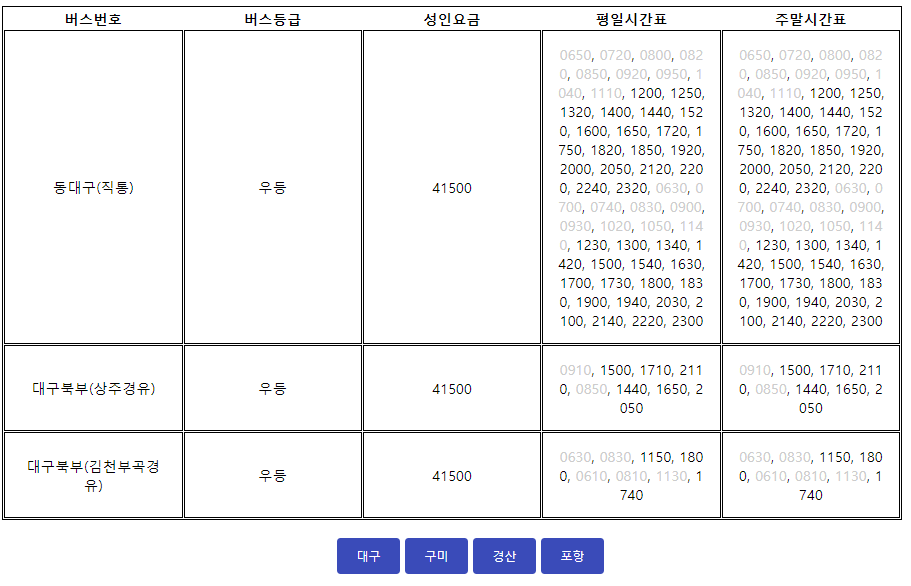
2. 대한항공 공공데이터
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
table {
border: 2px solid black;
empty-cells: hide;
width: 1000px;
table-layout: fixed;
word-break: break-all;
text-align: center;
}
td {
width: 200px;
height: 40px; /* 셀의 높이 */
padding: 15px;
border: 1px solid black;
text-align: center;
}
div {
margin: 0 auto;
padding: 20px;
text-align: center;
width: 1000px;
}
div input {
width: 70px;
height: 40px;
background-color: #3a4bb9;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
padding: 5px 10px;
}
div input:hover {
background-color: white;
color: #3a4bb9;
}
</style>
<script>
document.addEventListener('DOMContentLoaded', function () {
const btns = document.querySelectorAll('input[type=button]')
btns.forEach((item) => {
item.addEventListener('click', function () {
console.log(getUrl())
printAirPort(getUrl(), item.value)
})
})
})
const getUrl = function () {
const serviceKey = ''
const url = 'http://apis.data.go.kr/B551177/StatusOfPassengerFlightsDSOdp/getPassengerArrivalsDSOdp';
return `${url}?serviceKey=${serviceKey}&type=json`
}
const printAirPort = function (url,name) {
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.onreadystatechange = () => {
if (xhr.readyState === XMLHttpRequest.DONE) return;
if (xhr.status === 200) {
const jsonObject = JSON.parse(xhr.response)
const items = jsonObject.response.body.items;
const tableBody = document.querySelector('tbody');
while (tableBody.firstChild) {
tableBody.removeChild(tableBody.firstChild);
}
for (item of items) {
if (item["airport"].indexOf(name) >= 0) {
const trTag = document.createElement('tr')
trTag.innerHTML = `
<td>${item['airline']}</td>
<td>${item['flightId']}</td>
<td>${item['scheduleDateTime']}</td>
<td>${item['airport']}</td>
`;
document.body.append(trTag)
}
}
} else {
console.log('Error', xhr.status, xhr.statusText)
}
}
xhr.send()
}
</script>
</head>
<body>
<div>
<input type="button" value="나리타">
<input type="button" value="삿포로">
<input type="button" value="오사카">
<input type="button" value="후쿠오카">
</div>
<table>
<thead>
<tr>
<th>항공사</th>
<th>편명</th>
<th>예정시간</th>
<th>도착지공항</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
</body>
</html>
공부 과정을 정리한 것이라 내용이 부족할 수 있습니다.
부족한 내용은 추가 자료들로 보충해주시면 좋을 것 같습니다.
읽어주셔서 감사합니다 :)
'Dev > HTML CSS JS' 카테고리의 다른 글
JavaScript (Ajax, JSON, JSON.parse(), Ajax 이용 데이터 임포트, url 인코딩 키 사용) (2) | 2024.04.11 |
---|---|
Html Css JavaScript (Todo List, html, css, java script) (0) | 2024.04.09 |
Html Css JavaScript (수강신청 프로그램, html, css, 체크리스트 중간, html, css) (0) | 2024.04.08 |
JavaScript (참가신청 최종, 주문 프로그램, 회원가입) (2) | 2024.04.05 |
HTML CSS JS (연습문제, select, multiple, checkbox, radio, prevent, 참가신청) (0) | 2024.04.04 |