Learn & Record
Html Css JavaScript (Todo List, html, css, java script) 본문
1. Todo List
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link rel="stylesheet" href="./css/check_list.css">
</head>
<script>
/*
3. 등록시 조건
1) 글 등록이 된 후 폼 태그가 비워지도록
2) 할일과 날짜가 입력이 안된 경우 글 등록이 안되도록 (경고문을 발생하도록)
3) 날짜의 기본값은 오늘로
4) 이전 날짜는 선택이 안되도록
4. 글 삭제 기능 구현
1) 삭제 버튼에 이벤트 추가.
2) alert 창에 title 나오게
5) 글 수정 기능 구현
1) 수정 버튼 추가
*/
document.addEventListener('DOMContentLoaded', function () {
const title = document.querySelector('.add input[name=title]')
const dueDate = document.querySelector('.add input[name=dueDate]')
const btnAdd = document.querySelector('.add input[type=button]')
const list = document.querySelector('.list ul')
const btnDel = document.querySelector('input[name=delete]')
const editTit = document.querySelector('.edit input[name=title]')
const editDate = document.querySelector('.edit input[name=dueDate]')
const btnEdit = document.querySelector('.edit input[name=edit]')
dueDate.value = new Date().toISOString().slice(0,10)
dueDate.setAttribute('min', new Date().toISOString().slice(0,10))
btnAdd.addEventListener('click', function () {
if (title.value === '') {
alert('제목을 입력해주세요')
title.focus()
return
} else if (dueDate.value === '') {
alert('날짜를 선택해 주세요')
dueDate.focus()
return;
}
let addItem = document.createElement('li')
addItem.innerHTML = `<ul>\n
<li>${title.value}</li>\n\
<li>${dueDate.value}</li>\n
<li><input type="button" name="delete" value="x">
<input type="button" name="edit" value="수정"></li>\n
</ul>`;
addItem.querySelector('[name=delete]').addEventListener('click', function(event) {
console.log(event.currentTarget)
console.log(event.currentTarget.parentNode.parentNode.querySelector('li').textContent);
const userConfirmed = confirm(`${event.currentTarget.parentNode.parentNode.querySelector('li').textContent}를 삭제하시겠습니까?
* 수정 중이셨다면, 수정 영역이 초기화됩니다.`);
if (userConfirmed) {
// 3. 바로 삭제
const editTitle = document.querySelector('.edit input[name=title]')
const editDueDate = document.querySelector('.edit input[name=dueDate]')
const editIdx = document.querySelector('.edit input[name=idx]')
editTitle.value = ''
editDueDate.value = new Date().toISOString().slice(0,10)
editIdx.value = ''
addItem.remove()
}
// 1. removeChild() 사용.
// const node = event.currentTarget.parentNode.parentNode.parentNode; // li 삭제
// node.parentNode.removeChild(node)
// 2. remove() : 전체 요소에서 인덱스를 구해서 삭제
// 클릭한 부분의 인덱스 구함
// const list = document.querySelectorAll('.list ul')
// const array = Array.from(list); // 배열로 가져옴
// const indexNum = array.indexOf(event.currentTarget.parentNode.parentNode.parentNode); // 인덱스 구함
// // alert(indexNum);
//
// // 인덱스 기준으로 삭제
// array[indexNum].remove();
})
addItem.querySelector('[name=edit]').addEventListener('click', function (event) {
console.log(event.currentTarget)
const userConfirmed = confirm("수정하시겠습니까?");
if (userConfirmed) {
// 수정영역에 폼에 값을 입력
editTit.value = event.currentTarget.parentNode.parentNode.querySelector('li:nth-child(1)').textContent
editDate.value = event.currentTarget.parentNode.parentNode.querySelector('li:nth-child(2)').textContent
// 3. 해당 요소가 몇번째 인덱스인지 값을 입력
const list = document.querySelectorAll('.list ul')
const array = Array.from(list); // 배열로 가져옴
const indexNum = array.indexOf(event.currentTarget.parentNode.parentNode); // 인덱스 구함
document.querySelector('.edit [name=idx]').value = indexNum;
}
})
list.appendChild(addItem)
title.value = ''
dueDate.value = new Date().toISOString().slice(0,10)
// 수정영역에 있는 수정 버튼 클릭 시
btnEdit.addEventListener('click', function () {
const userConfirmed = confirm("수정을 완료하시겠습니까?")
const title = document.querySelector('.edit input[name=title]')
const dueDate = document.querySelector('.edit input[name=dueDate]')
const idx = document.querySelector('.edit input[name=idx]')
console.log(idx)
if (userConfirmed) {
const list = document.querySelectorAll('.list ul');
const array = Array.from(list); // 배열로 가져옴
console.log(array[idx.value]);
array[idx.value].querySelector('li:nth-child(1)').textContent = title.value;
array[idx.value].querySelector('li:nth-child(2)').textContent = dueDate.value;
}
})
})
})
</script>
<body>
<section class="list">
<h2>Todo</h2>
<ul>
<li>
<ul>
<li>할일</li>
<li>2023-12-31</li>
<li><input type="button" name="delete" value="x"></li>
</ul>
</li>
</ul>
</section>
<section class="add">
<h2>Add todo</h2>
<div class="addTool">
<input type="text" name="title">
<input type="date" name="dueDate">
<input type="button" value="등록">
</div>
</section>
<section class="edit">
<h2>Edit todo</h2>
<div class="addTool">
<input type="text" name="idx">
<input type="text" name="title">
<input type="date" name="dueDate">
<input type="button" name="edit" value="수정 ">
</div>
</section>
</body>
</html>
* {
margin: 0;
padding: 0;
}
body {
background: #ffffff;
}
h2 {
color: #004607;
text-shadow: 5px 5px 3px #a4a4a4;
}
section.list {
width: 500px;
margin: 10px auto;
padding: 15px;
margin-top: 40px;
background: #a1f19a;
border-radius: 10px;
height: 500px;
border: #185723 1px solid;
box-shadow: 5px 5px 3px #a4a4a4
}
section.add {
width: 500px;
margin: 10px auto;
padding: 15px;
margin-top: 40px;
background: #6fe36f;
border-radius: 10px;
border: #185723 1px solid;
box-shadow: 5px 5px 3px #9d9a9a
}
section.edit {
width: 500px;
margin: 10px auto;
padding: 15px;
margin-top: 40px;
background: #6fe36f;
border-radius: 10px;
border: #185723 1px solid;
box-shadow: 5px 5px 3px #9d9a9a
}
h2 {
margin-bottom: 20px;
}
.list ul li ul li {
display: inline;
text-align: center;
margin-left: 30px;
margin-right: 30px;
font-weight: bolder;
font-family: Pretendard;
font-size: 15px;
color: #185723;
text-shadow: 5px 5px 3px #a4a4a4;
}
.list ul li ul {
text-align: center;
border: #185723 solid 1px;
box-shadow: 5px 5px 3px #a4a4a4
}
.addTool {
text-align: center;
}
input[value='x'] {
background: #ff4848;
border: solid 1px #808080;
width: 20px;
height: 20px;
color: #000000;
border-radius: 100px;
font-family: Pretendard;
box-shadow: 5px 2px 3px #a4a4a4
}
input[value='등록'] {
background: #00ff77;
border: solid 1px black;
width: 70px;
height: 25px;
color: #000000;
border-radius: 5px;
font-family: Pretendard;
font-weight: bolder;
text-shadow: 5px 5px 3px #a4a4a4;
box-shadow: 5px 5px 3px #a4a4a4;
}
input[value='수정 '] {
background: #00a6ff;
border: solid 1px black;
width: 70px;
height: 25px;
color: #000000;
border-radius: 5px;
font-family: Pretendard;
font-weight: bolder;
text-shadow: 5px 5px 3px #a4a4a4;
box-shadow: 5px 5px 3px #a4a4a4;
}
input[value='수정'] {
background: #00a6ff;
border: solid 1px #ffffff;
width: 37px;
height: 20px;
color: #000000;
border-radius: 5px;
font-family: Pretendard;
font-weight: lighter;
text-shadow: 5px 5px 3px #a4a4a4;
box-shadow: 5px 2px 3px #a4a4a4;
margin-left: 10px;
}
.list ul {
background: #ebfde8;
height: 25px;
list-style: none;
margin-top: 10px;
border-radius: 5px;
}
input[type='text'] {
background: #ebfde8;
border: 1px solid black;
height: 22px;
border-radius: 8px;
font-weight: bolder;
font-family: Pretendard;
text-align: center;
text-shadow: 5px 5px 3px #a4a4a4;
box-shadow: 5px 5px 3px #a4a4a4
}
input[type='date'] {
background: #ebfde8;
border: 1px solid black;
height: 22px;
border-radius: 8px;
font-weight: bolder;
font-family: Pretendard;
text-align: center;
text-shadow: 5px 5px 3px #a4a4a4;
box-shadow: 5px 5px 3px #a4a4a4
}
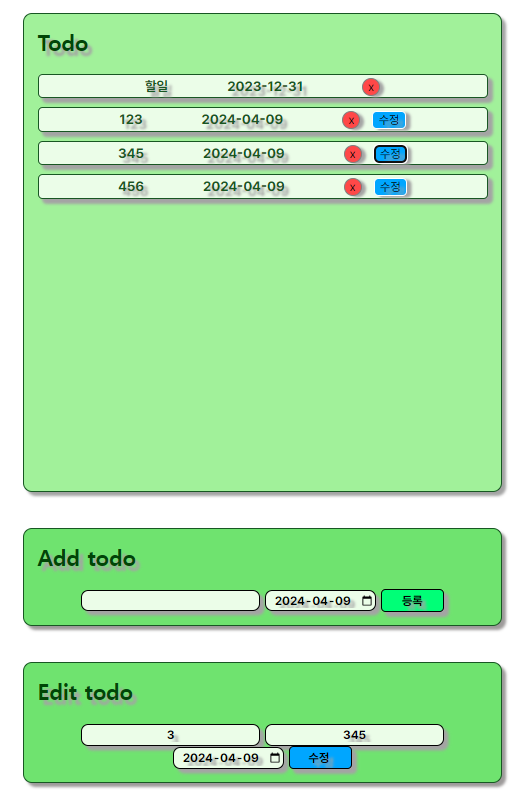
공부 과정을 정리한 것이라 내용이 부족할 수 있습니다.
부족한 내용은 추가 자료들로 보충해주시면 좋을 것 같습니다.
읽어주셔서 감사합니다 :)
'Dev > HTML CSS JS' 카테고리의 다른 글
JavaScript (인천국제공항_버스정보_ 공공데이터 활용, 여객편 주간 운항 공공데이터) (0) | 2024.04.12 |
---|---|
JavaScript (Ajax, JSON, JSON.parse(), Ajax 이용 데이터 임포트, url 인코딩 키 사용) (2) | 2024.04.11 |
Html Css JavaScript (수강신청 프로그램, html, css, 체크리스트 중간, html, css) (0) | 2024.04.08 |
JavaScript (참가신청 최종, 주문 프로그램, 회원가입) (2) | 2024.04.05 |
HTML CSS JS (연습문제, select, multiple, checkbox, radio, prevent, 참가신청) (0) | 2024.04.04 |