Learn & Record
HTML CSS JS (연습문제, select, multiple, checkbox, radio, prevent, 참가신청) 본문
HTML CSS JS (연습문제, select, multiple, checkbox, radio, prevent, 참가신청)
Walker_ 2024. 4. 4. 14:011. 연습문제
- 휴대폰 유효성 판별
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<script>
document.addEventListener('DOMContentLoaded', () => {
const input = document.querySelector('input');
const p = document.querySelector('p')
const isNumber = (value) => { // 휴대폰 번호 검사하는 함수
// 숫자 3자리 '-' 숫자 4자리 '-' 숫자 4자리
console.log(!isNaN(value.replaceAll('-','')))
console.log(value.split('-')[0] === '010')
// 숫자 3자리 '-' && 숫자 4자리 '-' && 숫자 4자리 '-' && 숫자를 입력했는지 && 010으로 시작하는지
return (value.split('-')[0].length == 3) && (value.split('-')[1].length == 4) && (value.split('-')[2].length == 4) && !isNaN(value.replaceAll('-','')) && value.split('-')[0]==='010';
};
input.addEventListener('keyup', function (event) {
const value = event.currentTarget.value;
console.log(value)
if (isNumber(value)) {
p.style.color = 'green'
p.textContent = `휴대폰 번호로 유효합니다. : ${value}`
}
else {
p.style.color = 'red'
p.textContent = `휴대폰 번호로 유효하지 않습니다. : ${value}`
}
})
})
</script>
<body>
<input type="text">
<p></p>
</body>
</html>
2. 폼태그 관련 Select
- 드롭다운 목록 활용하기
- 드롭다운 목록은 select 태그로 구현. 드롭 다운 목록을 선택했을 때(값이 변경 되었을 때) 어떤 것을 선택했는지 출력
- select 태그 Event = 'change'
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<script>
document.addEventListener('DOMContentLoaded', () =>{
const select = document.querySelector('select')
const p = document.querySelector('p')
select.addEventListener('change', (event)=> {
console.log(event)
const options = event.currentTarget.options; // option을 배열로 변환
const index = event.currentTarget.options.selectedIndex; // 선택한 index 추출
console.log(options[index].textContent)
p.textContent = `선택: ${options[index].textContent}`; // 선택한 option 태그를 추출
})
})
</script>
<body>
<select>
<option>떡볶이</option>
<option>순대</option>
<option>오뎅</option>
<option>튀김</option>
</select>
<p>선택: 떡볶이</p>
</body>
</html>
3. Multiple
- multiple select 태그 : select 태그에 multiple 속성을 부여하면 ctrl이나 shift를 누르고 복수의 항목을 선택 가능
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<script>
document.addEventListener('DOMContentLoaded', () =>{
const select = document.querySelector('select')
const p = document.querySelector('p')
select.addEventListener('change', (event)=> {
const options = event.currentTarget.options; // option을 배열로 변환
// console.log(options)
const list = [];
for (const option of options) { // options에는 forEach() 메소드가 없어서 for문을 돌림
console.log(option.selected)
if (option.selected) { // selected 속성 확인
list.push(option.textContent);
}
}
p.textContent = `선택: ${list.join(', ')}`
})
})
</script>
<body>
<select multiple>
<option>떡볶이</option>
<option>순대</option>
<option>오뎅</option>
<option>튀김</option>
</select>
<p>선택: 떡볶이</p>
</body>
</html>
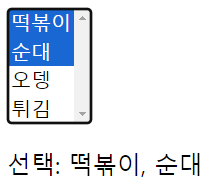
4. checkbox
- 이벤트 이름 : change
- 체크박스 활용하기
- 체크박스의 체크 상태를 확인할 때는 입력양식의 checked 속성을 사용
- 체크 상태일때만 타이머를 증가시키는 프로그램
- change 이벤트가 발생했을 때 체크 박스의 상태를 확인하고 setInterval() 함수 또는 clearInterval() 함수를 실행
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script>
document.addEventListener('DOMContentLoaded', () => {
const h1 = document.querySelector('h1')
const checkbox = document.querySelector('input')
let count = 0;
checkbox.addEventListener('change', (event)=> {
console.log(event.currentTarget.checked);
if (event.currentTarget.checked) { // 체크 상태
timeUp = setInterval(() => {
h1.textContent = `${count}초`
++count;
}, 1000)
} else { // 체크 해제 상태
clearInterval(timeUp)
h1.textContent = `${count}초`
}
})
})
</script>
</head>
<body>
<input type="checkbox">
<span>타이머 활성화</span>
<h1></h1>
</body>
</html>
5. 라디오 버튼 radiobutton
- 라디오버튼 활용하기
- 라디오 버튼은 name 속성이 동일하면 하나만 선택할 수 있음. 체크박스와 마찬가지로 checked 속성 사용
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script>
document.addEventListener('DOMContentLoaded', ()=>{
// 문서 객체 추출하기
const output = document.querySelector('#output')
const radios = document.querySelector('[name=pet]')
// 모든 라디오 버튼에
radios.forEach((radio) => {
// 이벤트 연결
radio.addEventListener('change', (event) => {
const current = event.currentTarget;
if (current.checked) {
output.textContent = `좋아하는 애완동물은 ${current.value}이시군요!`
}
});
});
});
</script>
</head>
<body>
<h3># 좋아하는 애완동물을 선택해주세요</h3>
<label><input type="radio" name="pet" value="강아지">
<span>강아지</span></label>
<label><input type="radio" name="pet" value="고양이">
<span>고양이</span></label>
<label><input type="radio" name="pet" value="햄스터">
<span>햄스터</span></label>
<label><input type="radio" name="pet" value="기타">
<span>기타</span></label>
<hr>
<h3 id="output"></h3>
</body>
</html>
6. 기본 이벤트 막기
- 기본 이벤트 : 어떤 이벤트가 발생했을 때 웹 브라우저가 기본적으로 처리해주는 것
- 기본 이벤트를 제거할 때는 event 객체의 preventDefault() 메소드를 사용
- 이미지 마우스 오른쪽 버튼 클릭 막기
- 웹 브라우저는 이미지에서 마우스 오른쪽 버튼을 클릭하면 컨텍스트 메뉴 context menu를 출력
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script>
// 기본 이벤트 막기
document.addEventListener('DOMContentLoaded', ()=>{
const imgs = document.querySelectorAll('img')
imgs.forEach((img) => {
img.addEventListener('contextmenu', (event)=> {
event.preventDefault(); // 컨텍스트 메뉴를 출력하는 기본 이벤트 제거
});
});
})
</script>
</head>
<body>
<img src="http://placebear.com/300/300" alt="">
</body>
</html>
7. preventDefault
- 체크 때만 링크 활성화 하기
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script>
// 기본 이벤트 막기
document.addEventListener('DOMContentLoaded', () => {
// 1. 페이지가 로딩된 후에 a 태그 링크 이벤트 막기
const link = document.querySelector('a');
const checkbox = document.querySelector('input');
let status = false;
checkbox.addEventListener('change', (event)=> {
status = event.currentTarget.checked;
})
link.addEventListener('click', (event) => {
if (!status){
event.preventDefault(); // status가 false가 아니면 링크의 기본 이벤트 제거
}
});
});
</script>
</head>
<body>
<input type="checkbox">
<span>링크 활성화</span>
<br>
<a href="http://naver.com">네이버</a>
</body>
</html>
8. 참가 신청
- 새 노드를 추가하고 표시하기
- input 태그에 문자열을 입력하고 [신청]버튼을 누르면, 리스트에 저장
- 추가시 맨위로 가도록 변경
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link rel="stylesheet" href="./css/name_list.css">
<script>
document.addEventListener('DOMContentLoaded', () => {
const btn = document.querySelector('button')
const userName = document.querySelector('#username')
const nameList = document.querySelector('#nameList')
btn.addEventListener('click', function (event){
// 기본 이벤트 (submit) 막기
event.preventDefault(); // submit 방지
// 1. 새로운 요소를 만들고, input 태그에 있는 문자열을 추가
// createElement() : 새로운 요소를 만듬
const item = document.createElement('p'); // 새 p 요소 만들기
item.textContent = userName.value;
// 2. nameList에 새로 만든 요소 추가하기
// nameList.appendChild(item); // p 요소를 element
nameList.insertBefore(item, nameList.childNodes[0]); // p요소를 #nameList 맨 앞에 추가하기
// 3. input태그에 문자열 제거하기
userName.value = ''; // 텍스트 필드 지우기
})
})
</script>
</head>
<body>
<div id="container">
<h1>참가 신청</h1>
<form action="">
<input type="text" id="username" placeholder="이름" required>
<button id="upLoad">신청</button>
</form>
<hr>
<div id="nameList"></div>
</div>
</body>
</html>
- - 코드 추가 작성 필요
공부 과정을 정리한 것이라 내용이 부족할 수 있습니다.
부족한 내용은 추가 자료들로 보충해주시면 좋을 것 같습니다.
읽어주셔서 감사합니다 :)